Hi everyone!
I'm Mario Horcajada, solo developer of the game Kronos. In this blog I will share my knowledge and experience of developing a 2D game with C++ and SFML.
First of all, learn a programming language (C++, Java...). Then take a look at the different graphic libraries out there. I recommend SFML, it's easy to start for begginers and has all the features you will need to make a complete 2D game.
I will cover most of the big topics from "Engine Design" to "AI Scripting".
If this blog helps anyone in his own game development, I will be happy :)
List of articles:
This is a minimum code extracted from SFML Tutorials, and shows how simple creating a window and drawing a shape can be:
#include <SFML/Graphics.hpp>
int main()
{
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!");
sf::CircleShape shape(100.f);
shape.setFillColor(sf::Color::Green);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
And the result:
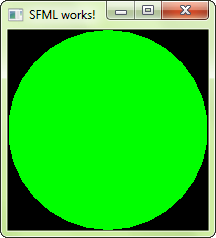
SDL and Allegro are good too. The best option? Try each one and decide for yourself which one match your needs.